In [1]:
1
2
from collections import Counter
from functools import reduce
Prime Factors
Prime Factor
Prime factor is the factor of the given number which is a prime number.
Factors are the numbers you multiply together to get another number.
In simple words, prime factor is finding which prime numbers multiply together to make the original number.
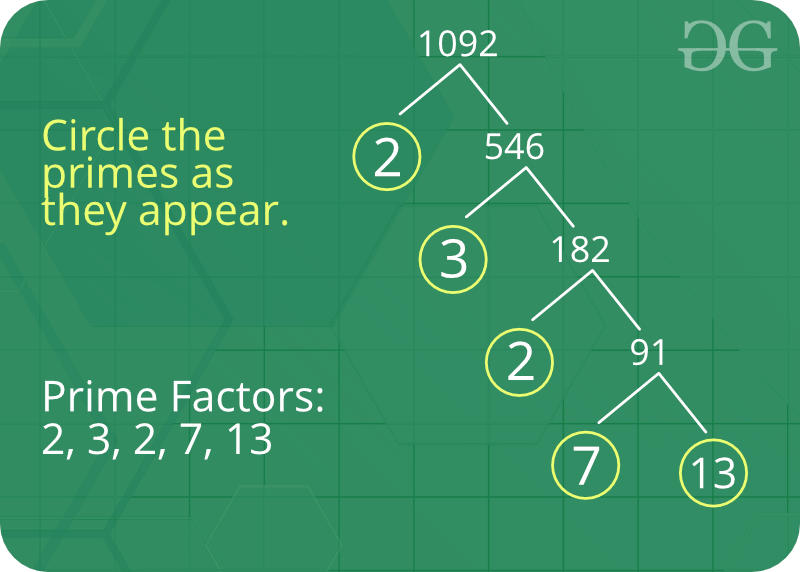
In [16]:
1
2
3
4
5
6
7
8
9
10
11
12
13
def prime_factors(n):
""" A function to print all prime factors of a given number n """
i = 2
factors = []
while i * i <= n:
if n % i: # if there is a remainder.
i += 1
else:
n //= i
factors.append(i)
if n > 1:
factors.append(n)
return factors
Find the number of divisors
If $10 = 2^2 \times 5^2$, the number of divisors as follows: $(2 + 1) \times (2 + 1) = 9$
In [22]:
1
2
3
4
5
def numOfDivs(n, verbose=False):
primes = Counter(prime_factors(n))
if verbose: print(primes)
cnts = list(map(lambda x : x + 1, primes.values()))
return reduce(lambda x, y: x * y, cnts)
In [23]:
1
numOfDivs(100, verbose=True)
1
2
Counter({2: 2, 5: 2})
1
9
Leave a comment